When you create a JHeadstart project, many files are automattically added to your project. One of those files is form.js which is a Javascript file. Open this file as it is where we'll be adding our Javascript code. This file is located in /jheadstart directory of the project.
Before I detail the code I added, there is a very helpful tip I found when using this file. If you want to see Javascript debug messages while testing your code, all you need to do is set the debug flag to true in form.js.
var debugMode = true;
Update Form.js
Now, back to the task at hand which is adding confirm delete functionality to your table layout. Add the following code to form.js:
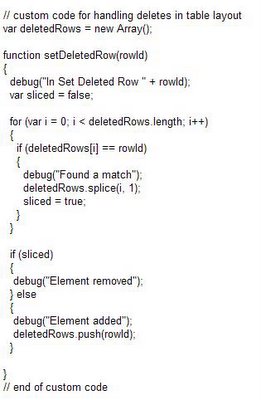
The deletedRows array is used to track what rows have been selected for deletion. The setDeletedRow function is used to add and remove elements from this array. If there is nothing in the array, then the user didn't select anything otherwise we'll know we need to prompt the user.
That is all the changes we need to make to form.js. The other changes we'll need to make directly to our UIX pages. We'll be adding additional Javascript and onClick events to the Save button and checkboxes.
Add Javascript
We'll need to add a Javascript function to the UIX page to handle clicking on the Save button. Following is the code to be added:
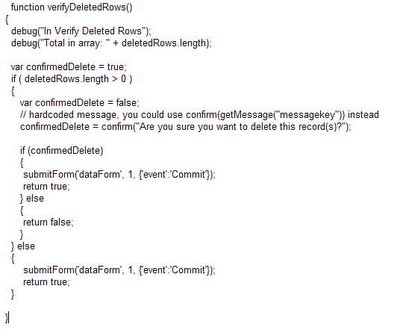
Add checkbox onClick event
Select the checkbox in the design editor. Open the property inspector and add the following to the onClick event:
setDeletedRow(${uix.current.PrimaryKeyId});
You'll need to replace PrimaryKeyId with a unique value from your VO. This id is added or removed from the Javascript array and is how the system can determine if at least one row has been checked.
Change Save button
By default, JHeadstart generates a Save button similar to the following:
<submitbutton onclick="verifyDeletedRows();" event="Commit" formname="dataForm" textandaccesskey="${nls.WHATEVER}";>
We'll need to change that so that it calls our Javascript code we added above. Following is the new updated Save buton:
<button onclick="return verifyDeletedRows();" textandaccesskey="${nls.WHATEVER}">
Run the app
After you get those steps completed successfully, you should see something like this when you execute your program:
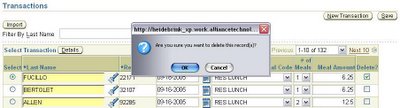
Last note, make sure to follow this to ensure you document your post generation changes so that you can continue to generate your app. You can also specify at a page level in JHS which page(s) for the generator to ignore.