As the user makes changes to either the type or quantity field, I also wanted the system to perform the update without refreshing the entire page as this is a web application. I wanted this calculation to happen in either table layout or form layout. I was able to meet all of these requirements using JHeadstart and Partial Page Rendering functionality in JDeveloper.
Following are "partial" steps to set this up. I say partial because these steps are only if you are needing the change to fire based on one field change instead of two (as in my scenario). I have also created an example based upon the SCOTT schema that I can send anyone. Drop me a line at heidebrinks@alliancechnologies.net and I'll send it to you.
All of these steps are completed AFTER your application has been generated using JHeadstart 10.1.2.
- Create a new struts action that extends JhsDataAction and add a method to handle the event.
- We'll want this struts action to work for both table and form layout. Add code to this method to grab the currently "changed" row. The "layoutType" and "rowIndex" parameters we'll be setting in a later step.
- Add code to the "onWhenValChanged" method to execute the necessary business rule(s). This code is best maintained in the business services layer and simply called from here.
- Open the UIX page that is in form layout. Using the design editor, select the field you are wanting to fire the change. With the field selected, open the Property Inspector and select "primaryClientAction". The following image is an example. Notice the use of the "layoutType" parameter. Set this to "F" to indicate form. The "Action Event" property is set to "whenValChanged" which will call our method "onWhenValChanged" in the JhsDataAction we created.
- Open the UIX page that is in table layout. Using the design editor, select the field you are wanting to fire the change. With the field selected, open the Property Inspector and select "primaryClientAction". The following image is an example. Notice the use of "layoutType" and "rowIndex" parameter. The "rowIndex" parameter is used by the JhsDataAction to determine which row is being changed by the user in the table layout.
- For the page that is in table layout ONLY, open the source editor. Update multiRowUpdateEvent to include the new event you are creating.
formValue name="multiRowUpdateEvent"
value="whenValChanged" - Update struts config so that both actions for the form and table use the new JhsDataAction.
public void onWhenValChanged(DataActionContext ctx) {
}
public void onWhenValChanged(DataActionContext ctx) {
// layout type will determine how we access the row
String layoutTypeParam = ctx.getHttpServletRequest().getParameter("layoutType");
Row row;
if (layoutTypeParam.equalsIgnoreCase("T")) {
// since this is table layout, we need to get the row index to know which one
String rowIndexParam = ctx.getHttpServletRequest().getParameter("rowIndex");
row = ctx.getBindingContainer()
.findIteratorBinding("EmpIterator")
.getNavigatableRowIterator().getRowAtRangeIndex(Integer.parseInt(
rowIndexParam));
} else {
row = ctx.getBindingContainer()
.findIteratorBinding("EmpIterator")
.getNavigatableRowIterator().getCurrentRow();
}
}
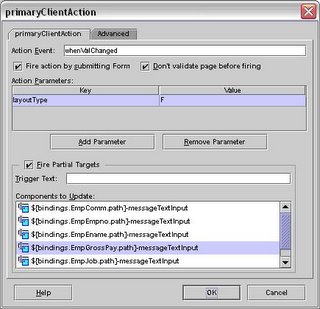
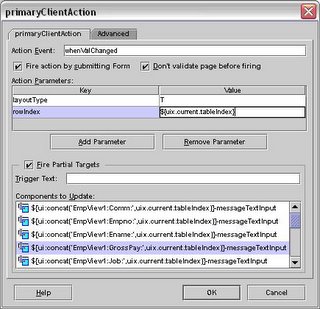
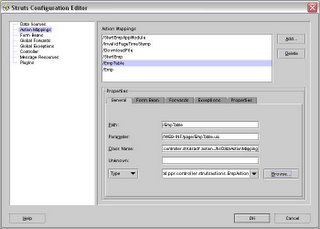
Just let me know if you want the example application I developed. It should be helpful if you are trying to implement similar functionality.
No comments:
Post a Comment